Use TcpClient with SslStream to request an HTTPS URL (And add your own headers!)
11/9/2021
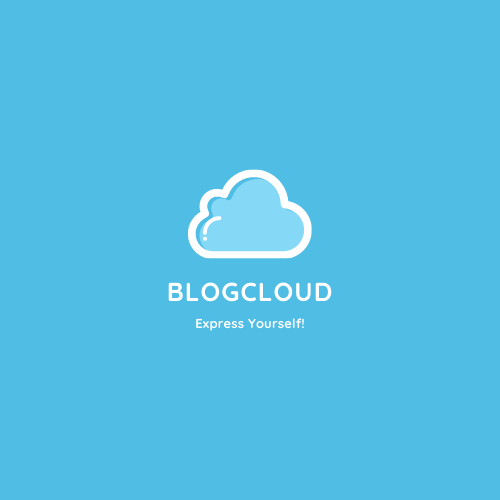
Author
Gal RatnerYou can use a Socket to request any http URL on the web just by sending your own HTTP formatted request, but what about https URLs?
To programmatically request a secure URL over SSL we will need to use an SslStream stream and negotiate the exchange od certificates.
First lets construct the request we will be sending to the remote server over port 443.
string host = "www.76psychics.com"; string path = "/Psychic/lucinda"; int port = 443; string request = "GET "+ path + " HTTP/1.1\r\nHost: " + host + "\r\n" + "Referer: Gal Ratner's Blog\r\n" + "User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/95.0.4638.69 Safari/537.36\r\n" + "Accept-Encoding: deflate\r\n" + "Connection: close\r\n\r\n";
The code to send the request and write back the response is:
public static void TcpClientSendReceive(String server, int port, String message) { using (TcpClient client = new TcpClient(server, port)) { using (SslStream sslStream = new SslStream(client.GetStream(), false)) { try { sslStream.AuthenticateAsClient(server); Byte[] data = System.Text.Encoding.ASCII.GetBytes(message); sslStream.Write(data, 0, data.Length); sslStream.Flush(); Console.WriteLine("Sent: {0}", message); string responseData = String.Empty; using (StreamReader sr = new StreamReader(sslStream)) { responseData = sr.ReadToEnd(); } Console.WriteLine($"Received: {responseData}"); sslStream.Close(); client.Close(); } catch (ArgumentNullException e) { Console.WriteLine("ArgumentNullException: {0}", e); } catch (SocketException e) { Console.WriteLine("SocketException: {0}", e); } catch (Exception e) { Console.WriteLine("Exception: {0}", e); } } } }
Finally lets call our function:
Console.WriteLine($"Requesting URL {host}"); GetSocket.TcpClientSendReceive(host, port, request);
Did you find this post useful? Please consider visiting my software consulting firm Inverted Software
We provide quality custom software that is on time and on budget.
Related Tags:
2 Comments.
Leave a CommentMike
8/7/2022Amazing!
Madison Jones
11/15/2021Thank you for the tutorial. Saved me hours of research!