Logging To a File or Database in .NET Core and ASP.NET Core
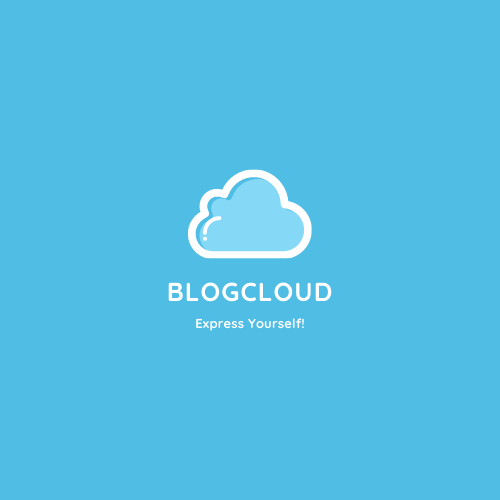
Author
Gal RatnerNET Core’s architecture supports a logging API that works with a variety of built-in and third-party logging providers. This article will explore using a provider in order to log into a file or a database.
The default Logging Providers included in .NET Core are:
- Console
- Debug
- EventSource
- EventLog (only when running on Windows)
Which only partially cover most logging use cases.
By adding PLogger from Inverted Software, you can add file and database logging capability to your application.
To add PLogger, add the following Nuget Package.
Once the package is added, change your CreateHostBuilder method to something like this:
public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureLogging((hostingContext, logging) => { logging.ClearProviders(); logging.AddPLogger(); logging.SetMinimumLevel(LogLevel.Information); }) .ConfigureWebHostDefaults(webBuilder => { webBuilder.UseStartup<Startup>(); });
In your Configure method, inject an ILoggerFactory as a parameter and and add PLogger with an IConfiguration object:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env, ILoggerFactory loggerFactory) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseHttpsRedirection(); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); loggerFactory.AddPLogger(Configuration); }
PLogger needs a configuration section in your appsettings.json file.
This typical PLogger configuration:
{ "Logging": { "LogLevel": { "Default": "Information", "Microsoft": "Warning", "Microsoft.Hosting.Lifetime": "Information" }, "PLogger": { "PLogType": "file", "PLogEnabled": true, "BaseNameFile": "plogger.txt", "PLogFileMaxSizeKB": 1024, "PLogFileMessageTemplate": "{0}", "StringConnection": "StringConnection", "StoredProcedureName": "AddError", "MessageParameterName": "@ErrorName" } }, "AllowedHosts": "*" }
Full configuration options are on the project’s GutHub repository here.
Once you configured your logger, you can then start using it in your application.
[HttpGet] [ProducesResponseType(StatusCodes.Status200OK)] [ProducesResponseType(StatusCodes.Status500InternalServerError)] public ActionResult<IEnumerable<WeatherForecast>> Get() { _logger.LogInformation("Callled Get"); try { var rng = new Random(); _logger.LogInformation("Generating Response"); var myResult = Enumerable.Range(1, 5).Select(index => new WeatherForecast { Date = DateTime.Now.AddDays(index), TemperatureC = rng.Next(-20, 55), Summary = Summaries[rng.Next(Summaries.Length)] }) .ToArray(); _logger.LogInformation("Generated results with " + myResult.Length + " elements"); return myResult; } catch (Exception ex) { _logger.LogError(ex, "Error in Get"); return StatusCode(StatusCodes.Status500InternalServerError); } }
Enjoy!